Slack Driven Development¶
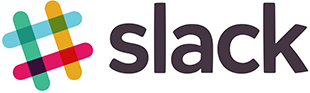
Date: 2016-06-15
Since I am always sitting in a slack channel, I wanted to share a tool I use to track exceptions across my cloud and other environments.
This repository is an example for posting: exceptions, tracking environment errors, and sending targeted debugging messages to a Slack channel to help decrease time spent finding + fixing bugs.
I built this because I was tired of tail-ing and grep-ing through logs. Now I just wait for exceptions and errors to be published into a debugging Slack channel so I can quickly view: what environment
had the error, the associated message
, and the source code line number
.
Setup¶
This repository only works with Python 2.
Install the official Slack Python API
Note
Please refer to the official Slack repository for more information
sudo pip install slackclient
Getting Started¶
Create a Slack channel for debugging messages
This demo uses a channel with the name:
#debugging
Create a named Slack bot if you do not have one already
This demo uses a bot with the name:
bugbot
Record the Slack bot’s API Token
This demo uses a bot with an API token:
xoxb-51351043345-oHKTiT5sXqIAlxwYzQspae54
Invite the Slack bot to your debugging channel
Type this into the debugging channel and hit enter:
/invite bugbot
Warning
If you do not invite the bot into the channel it can be difficult to debug
Edit the configuration for your needs
The example file slack_driven_development.py uses a configuration dictionary object where you can assign these keys based off your needs:
Key Name
Purpose and Where to find the Value
BotName
Name of the Slack bot that will post messages
ChannelName
Name of the channel where messages are posted
NotifyUser
Name of the Slack user that gets notified for messages
Token
Slack API Token for the bot
EnvName
A logical name for showing which environment had the error
Test the bot works
The included slack_driven_development.py file will throw an exception that will report the exception into the channel using the bot based off the simple dictionary configuration in the file.
$ ./slack_driven_development.py Testing an Exception that shows up in Slack Sending an error message to Slack for Exception(name 'testing_how_this_looks_from_slack' is not defined) Done $
Confirm the debugging message shows up in Slack channel
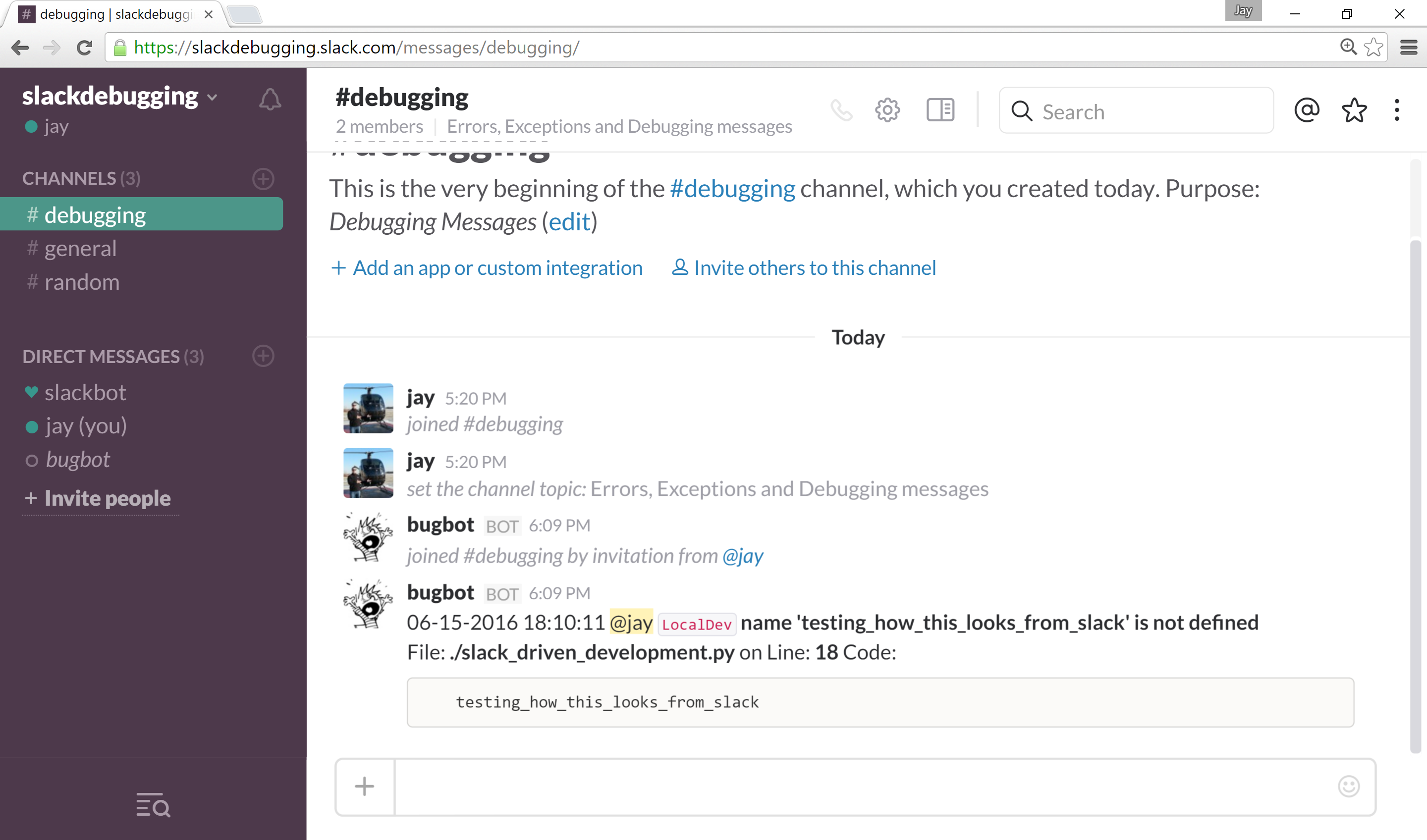
Screenshots for Getting Started¶
Here are the screenshots (as of 06-15-2016) for getting this demo integrated with your Slack channel and bot:
1. Create a new Slack App Integration
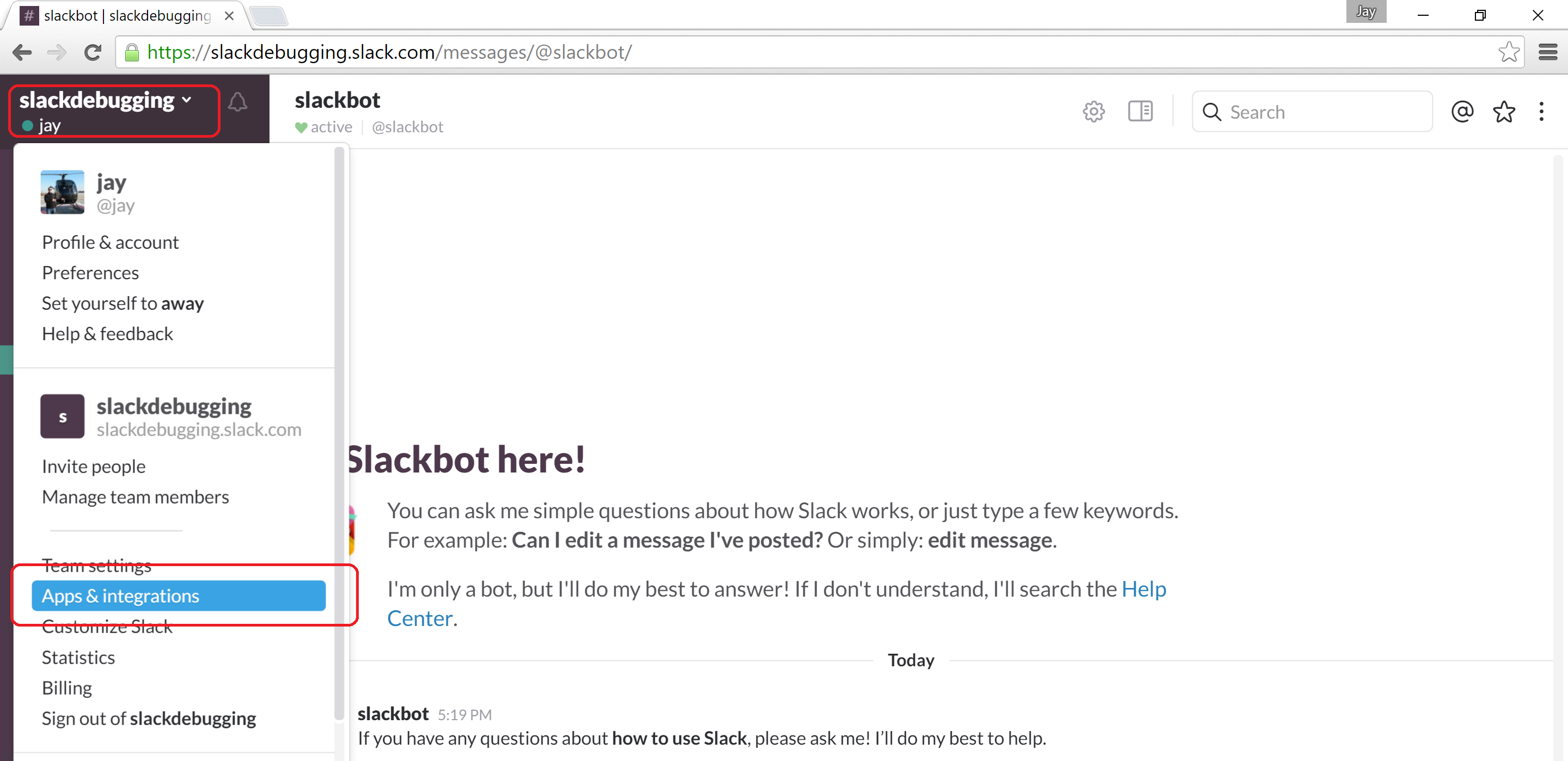
2. Build a new Integration
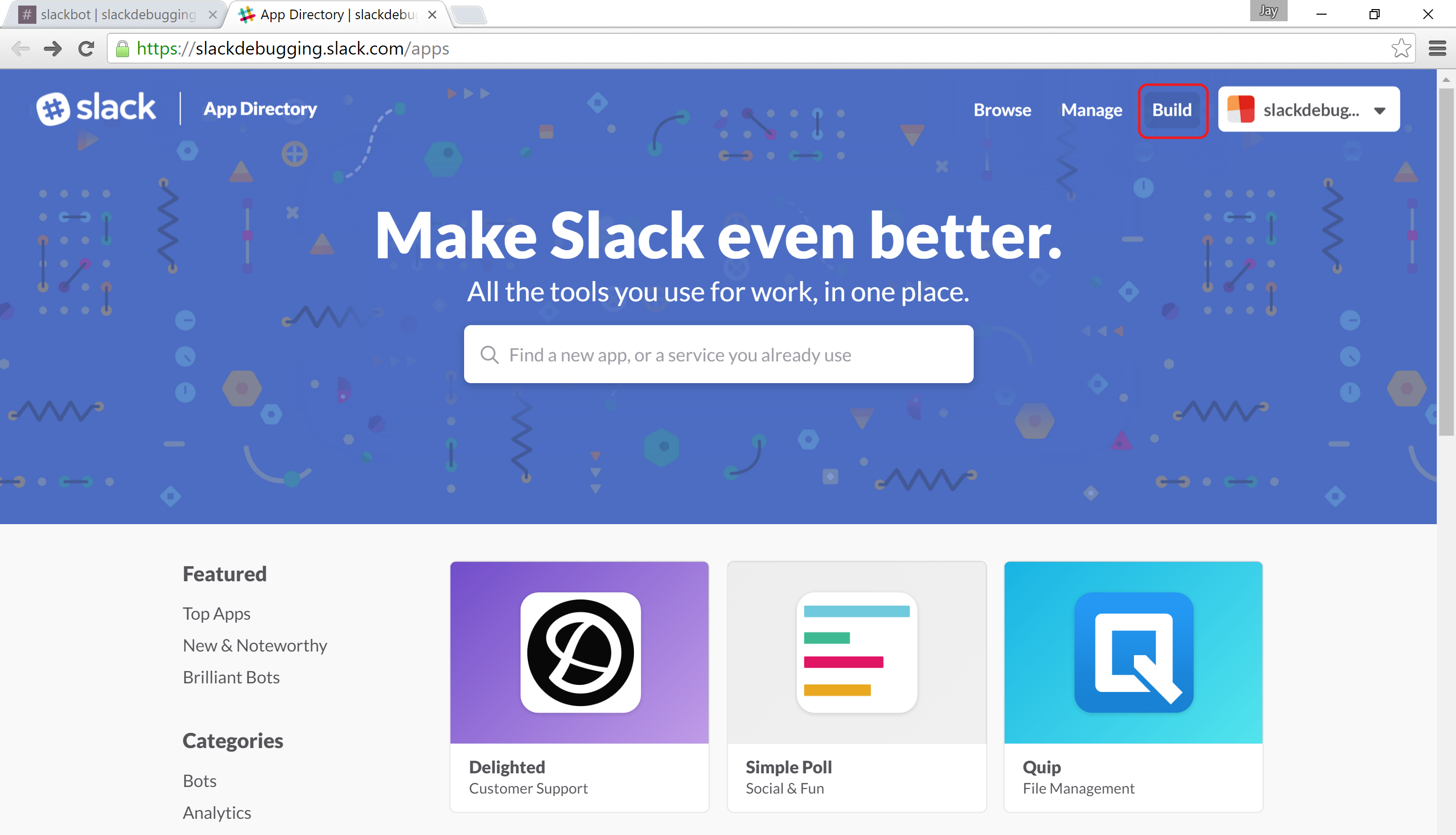
3. Make a Custom Integration
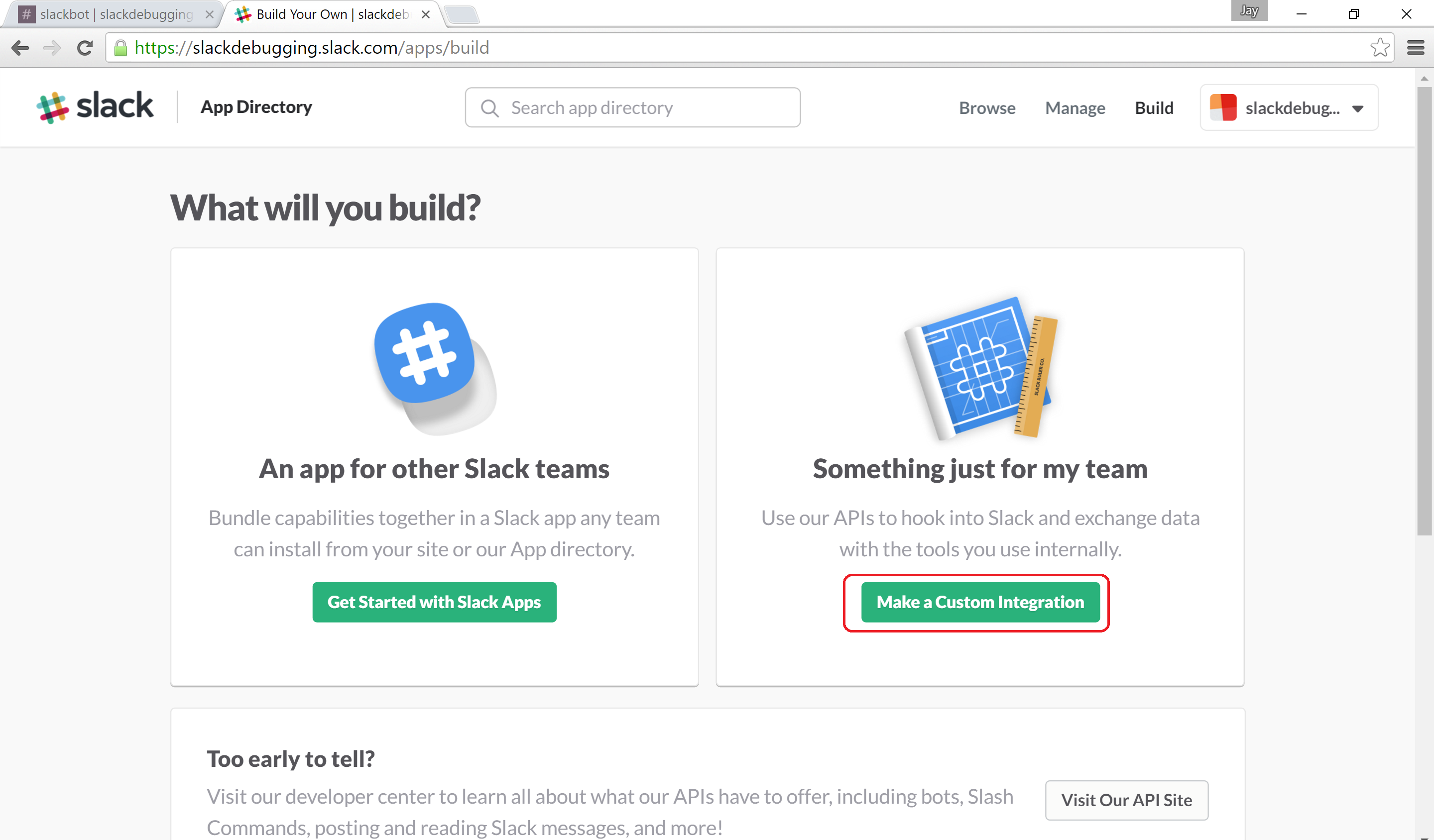
4. Create a new Bot
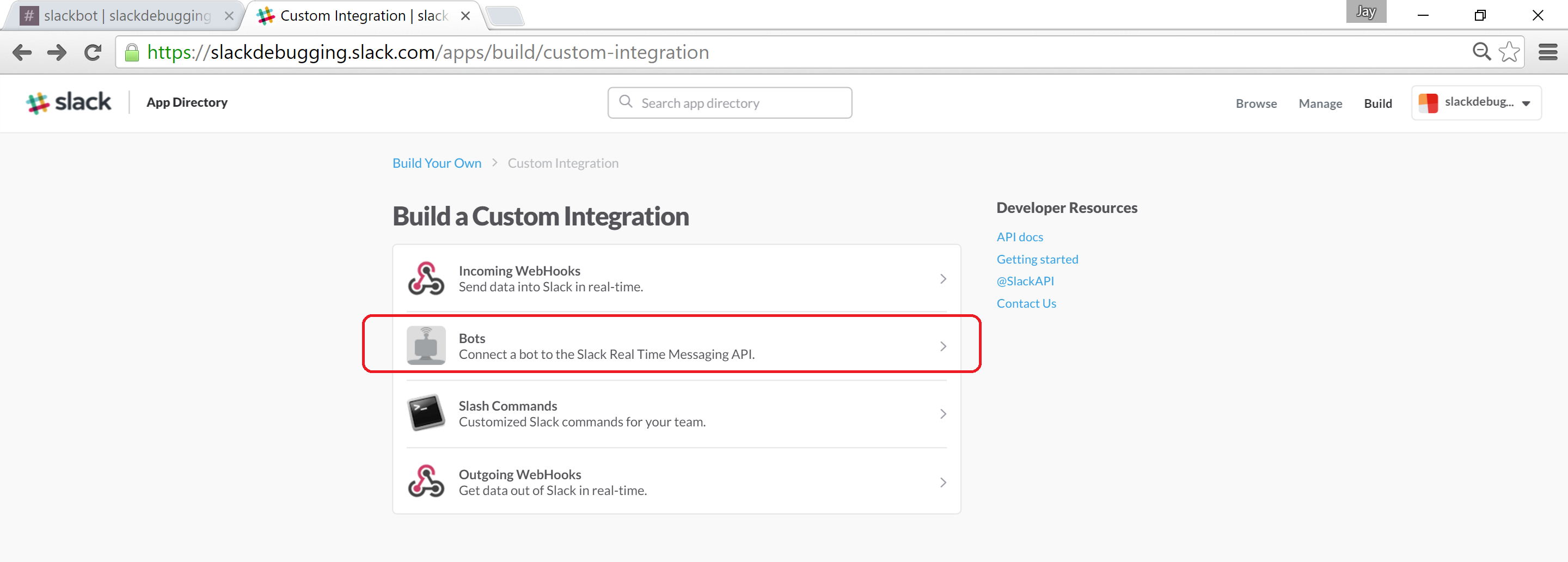
5. Name and Add Bot
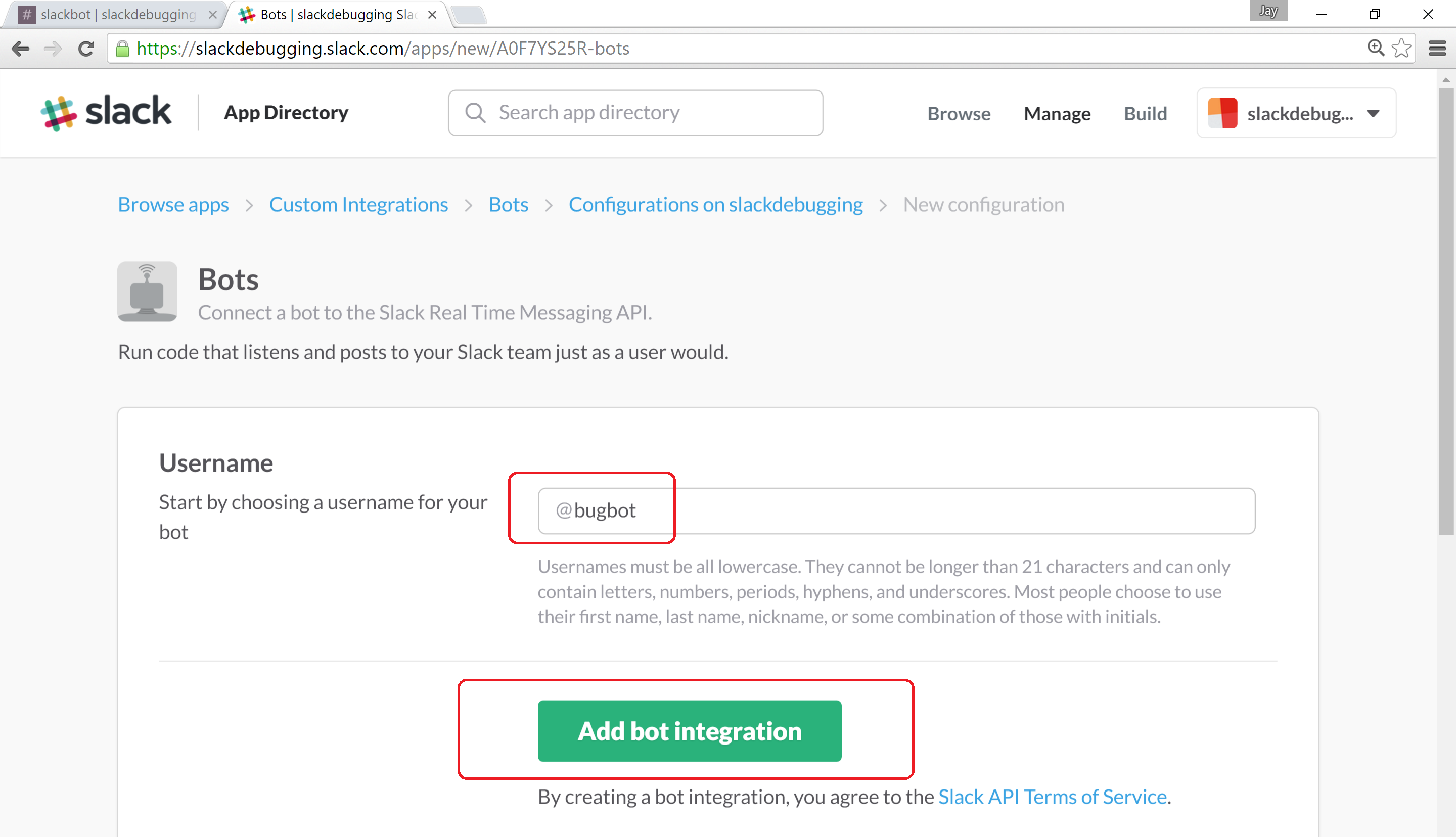
6. Record the Bot API Token in the Dictionary Configuration
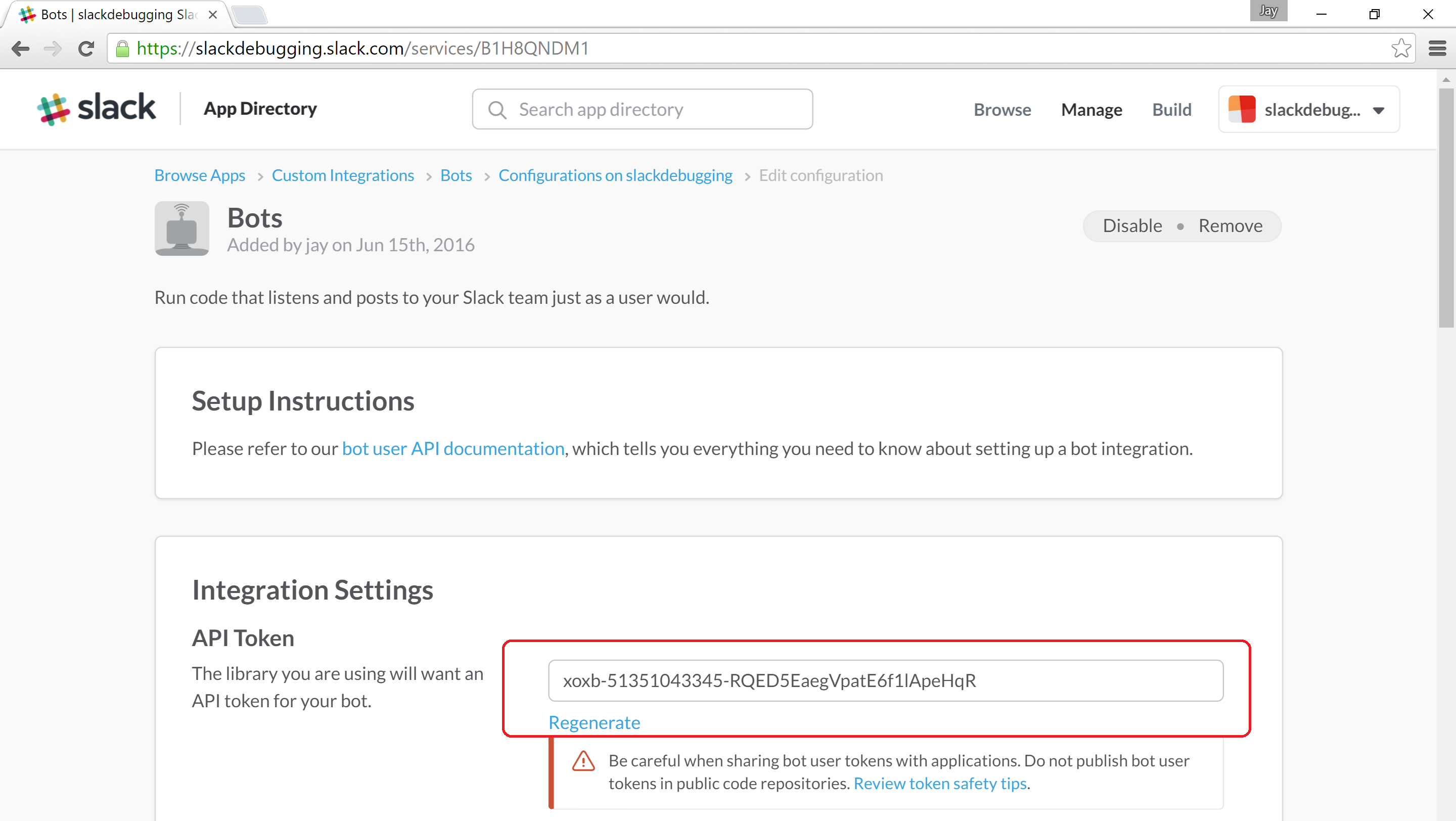
7. Run the Slack Driven Development Demo
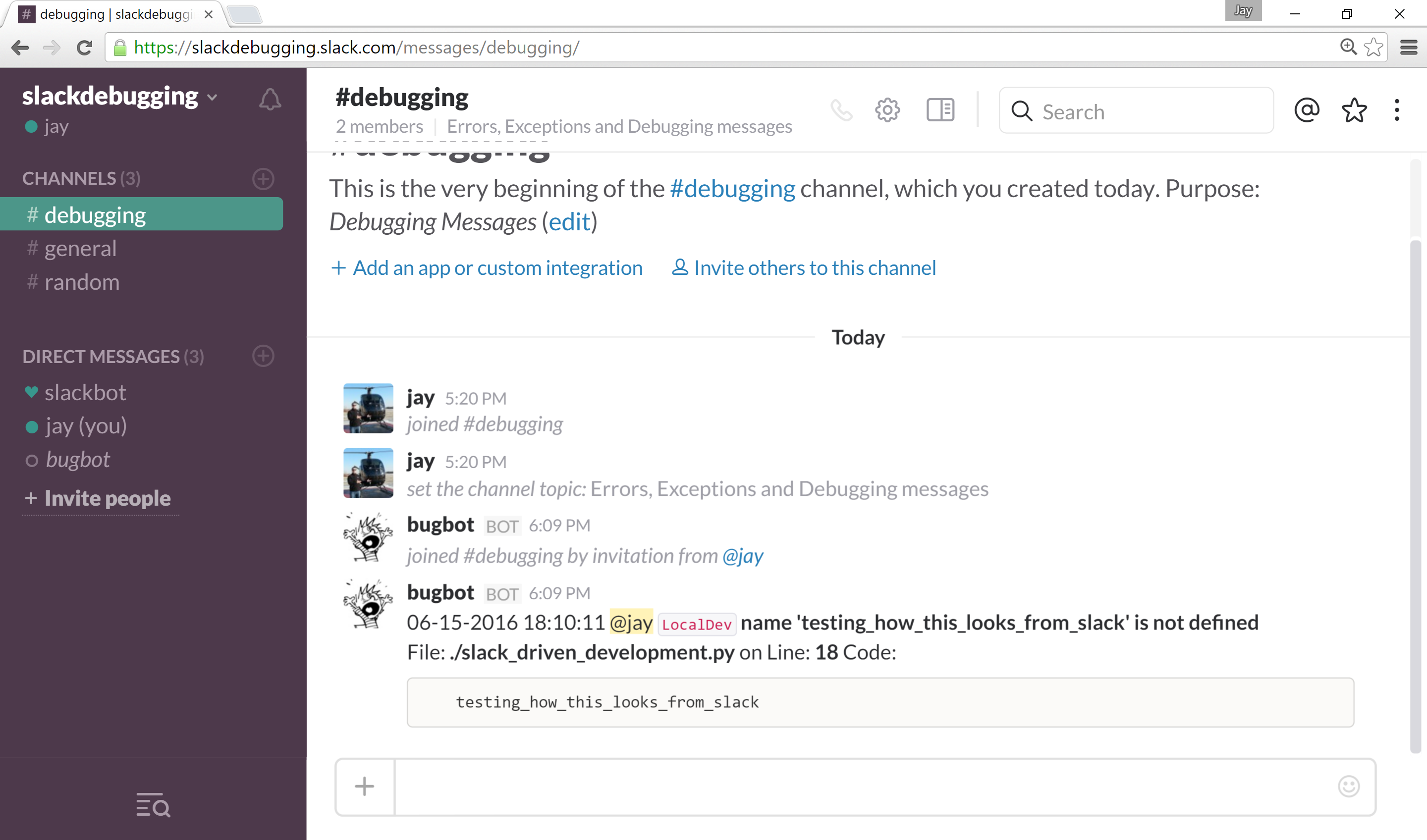
How does this work?¶
By using a try/catch block we can capture the exception, and pass it into a handler method.
1try: 2 print "Testing an Exception that shows up in Slack" 3 testing_how_this_looks_from_slack 4except Exception,k: 5 print "Sending an error message to Slack for the expected Exception(" + str(k) + ")" 6 slack_msg.handle_send_slack_internal_ex(k) 7# end of try/ex
-
Python has a great little feature where it can inspect the stacktrace from an exception outside of the calling objects and even files. Once the file with the exception has been found, we can open the file and goto the exact line that caused the exception and build an error report with the source code and the line number.
1def get_exception_error_message(self, ex, exc_type, exc_obj, exc_tb): 2 3 path_to_file = str(exc_tb.tb_frame.f_code.co_filename) 4 last_line = int(exc_tb.tb_lineno) 5 gh_line_number = int(last_line) - 1 6 file_name = str(os.path.split(exc_tb.tb_frame.f_code.co_filename)[1]) 7 path_to_file = str(exc_tb.tb_frame.f_code.co_filename) 8 py_file = open(path_to_file).readlines() 9 line = "" 10 for line_idx,cur_line in enumerate(py_file): 11 if line_idx == gh_line_number: 12 line = cur_line 13 14 if str(exc_obj.message) != "": 15 ex_details_msg = str(exc_obj.message) 16 else: 17 ex_details_msg = str(ex) 18 19 send_error_log_to_slack = "" 20 if line != "": 21 send_error_log_to_slack = " File: *" + str(path_to_file) + "* on Line: *" + str(last_line) + "* Code: \n```" + str(line) + "``` \n" 22 else: 23 send_error_log_to_slack = "Error on Line Number: " + str(last_line) 24 25 return send_error_log_to_slack 26# end of get_exception_error_message
Send the message to the Slack channel
Post the message to the Slack channel and handle any exceptions.
Note
If your configuration dictionary object is misconfigured you will likely see an
unauthorized
error
License¶
This repository is licensed under the MIT license.
Thanks for reading,
Jay